Note
Go to the end to download the full example code.
Catalytic combustion of methane on platinum#
This script solves a catalytic combustion problem. A stagnation flow is set up, with a gas inlet 10 cm from a platinum surface at 900 K. The lean, premixed methane/air mixture enters at ~6 cm/s (0.06 kg/m2/s), and burns catalytically on the platinum surface. Gas-phase chemistry is included too, and has some effect very near the surface.
The catalytic combustion mechanism is from Deutschmann et al., 26th Symp. (Intl.) on Combustion,1996 pp. 1747-1754
Requires: cantera >= 3.0, matplotlib >= 2.0
import numpy as np
import matplotlib.pyplot as plt
import cantera as ct
Problem Definition#
Parameter values are collected here to make it easier to modify them
We will solve first for a hydrogen/air case to use as the initial estimate for the methane/air case
# composition of the inlet premixed gas for the hydrogen/air case
comp1 = 'H2:0.05, O2:0.21, N2:0.78, AR:0.01'
# composition of the inlet premixed gas for the methane/air case
comp2 = 'CH4:0.095, O2:0.21, N2:0.78, AR:0.01'
# The inlet/surface separation is 10 cm.
width = 0.1 # m
loglevel = 1 # amount of diagnostic output (0 to 5)
Create the phase objects#
The surf_phase
object will be used to evaluate all surface chemical production
rates. It will be created from the interface definition Pt_surf
in input file
ptcombust.yaml
, which implements the reaction mechanism of Deutschmann et
al., 1995 for catalytic combustion on platinum.
This phase definition also references the phase gas
in the same input file,
which will be created and used to evaluate all thermodynamic, kinetic, and
transport properties. It is a stripped-down version of GRI-Mech 3.0.
surf_phase = ct.Interface("ptcombust.yaml", "Pt_surf")
surf_phase.TP = tsurf, p
gas = surf_phase.adjacent["gas"]
gas.TPX = tinlet, p, comp1
# integrate the coverage equations in time for 1 s, holding the gas
# composition fixed to generate a good starting estimate for the coverages.
surf_phase.advance_coverages(1.0)
# create the object that simulates the stagnation flow, and specify an initial
# grid
sim = ct.ImpingingJet(gas=gas, width=width, surface=surf_phase)
# Objects of class ImpingingJet have members that represent the gas inlet
# ('inlet') and the surface ('surface'). Set some parameters of these objects.
sim.inlet.mdot = mdot
sim.inlet.T = tinlet
sim.inlet.X = comp1
sim.surface.T = tsurf
Show the initial solution estimate
sim.show()
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>> inlet <<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
Mass Flux: 0.06 kg/m^2/s
Temperature: 300 K
Mass Fractions:
H2 0.003467
O2 0.2311
AR 0.01374
N2 0.7516
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>> flame <<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
Pressure: 1.013e+05 Pa
-------------------------------------------------------------------------------
z velocity spread_rate T lambda eField
-------------------------------------------------------------------------------
0 0.05335 0 300 0 0
0.02 0.04268 0 420 0 0
0.04 0.03201 0 540 0 0
0.06 0.02134 0 660 0 0
0.08 0.01067 0 780 0 0
0.1 0 0 900 0 0
-------------------------------------------------------------------------------
z Uo H2 H O O2
-------------------------------------------------------------------------------
0 0 0.003467 0 0 0.2311
0.02 0 0.003467 0 0 0.2311
0.04 0 0.003467 0 0 0.2311
0.06 0 0.003467 0 0 0.2311
0.08 0 0.003467 0 0 0.2311
0.1 0 0.003467 0 0 0.2311
-------------------------------------------------------------------------------
z OH H2O HO2 H2O2 C
-------------------------------------------------------------------------------
0 0 0 0 0 0
0.02 0 0 0 0 0
0.04 0 0 0 0 0
0.06 0 0 0 0 0
0.08 0 0 0 0 0
0.1 0 0 0 0 0
-------------------------------------------------------------------------------
z CH CH2 CH2(S) CH3 CH4
-------------------------------------------------------------------------------
0 0 0 0 0 0
0.02 0 0 0 0 0
0.04 0 0 0 0 0
0.06 0 0 0 0 0
0.08 0 0 0 0 0
0.1 0 0 0 0 0
-------------------------------------------------------------------------------
z CO CO2 HCO CH2O CH2OH
-------------------------------------------------------------------------------
0 0 0 0 0 0
0.02 0 0 0 0 0
0.04 0 0 0 0 0
0.06 0 0 0 0 0
0.08 0 0 0 0 0
0.1 0 0 0 0 0
-------------------------------------------------------------------------------
z CH3O CH3OH C2H C2H2 C2H3
-------------------------------------------------------------------------------
0 0 0 0 0 0
0.02 0 0 0 0 0
0.04 0 0 0 0 0
0.06 0 0 0 0 0
0.08 0 0 0 0 0
0.1 0 0 0 0 0
-------------------------------------------------------------------------------
z C2H4 C2H5 C2H6 HCCO CH2CO
-------------------------------------------------------------------------------
0 0 0 0 0 0
0.02 0 0 0 0 0
0.04 0 0 0 0 0
0.06 0 0 0 0 0
0.08 0 0 0 0 0
0.1 0 0 0 0 0
-------------------------------------------------------------------------------
z HCCOH AR N2
-------------------------------------------------------------------------------
0 0 0.01374 0.7516
0.02 0 0.01374 0.7516
0.04 0 0.01374 0.7516
0.06 0 0.01374 0.7516
0.08 0 0.01374 0.7516
0.1 0 0.01374 0.7516
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>> surface <<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
Temperature: 900 K
Coverages:
PT(S) 0.4879
H(S) 4.738e-06
H2O(S) 2.755e-05
OH(S) 0.01028
CO(S) 0
CO2(S) 0
CH3(S) 0
CH2(S)s 0
CH(S) 0
C(S) 0
O(S) 0.5018
Solving problems with stiff chemistry coupled to flow can require a sequential approach where solutions are first obtained for simpler problems and used as the initial guess for more difficult problems.
# disable the surface coverage equations, and turn off all gas and surface
# chemistry.
sim.surface.coverage_enabled = False
surf_phase.set_multiplier(0.0)
gas.set_multiplier(0.0)
solve the problem, refining the grid if needed, to determine the non- reacting velocity and temperature distributions
************ Solving on 6 point grid with energy equation enabled ************
..............................................................................
Attempt Newton solution of steady-state problem.
Newton steady-state solve succeeded.
Problem solved on [6] point grid(s).
..............................................................................
grid refinement disabled.
******************** Solving with grid refinement enabled ********************
..............................................................................
Attempt Newton solution of steady-state problem.
Newton steady-state solve succeeded.
Problem solved on [6] point grid(s).
..............................................................................
##############################################################################
Refining grid in flame.
New points inserted after grid points 3 4
to resolve T spread_rate
##############################################################################
..............................................................................
Attempt Newton solution of steady-state problem.
Newton steady-state solve succeeded.
Problem solved on [8] point grid(s).
..............................................................................
##############################################################################
Refining grid in flame.
New points inserted after grid points 5 6
to resolve spread_rate
##############################################################################
..............................................................................
Attempt Newton solution of steady-state problem.
Newton steady-state solve succeeded.
Problem solved on [10] point grid(s).
..............................................................................
no new points needed in flame
now turn on the surface coverage equations, and turn the chemistry on slowly
sim.surface.coverage_enabled = True
for mult in np.logspace(-5, 0, 6):
surf_phase.set_multiplier(mult)
gas.set_multiplier(mult)
print('Multiplier =', mult)
sim.solve(loglevel)
Multiplier = 1e-05
..............................................................................
Attempt Newton solution of steady-state problem.
Newton steady-state solve succeeded.
Problem solved on [10] point grid(s).
..............................................................................
no new points needed in flame
Multiplier = 0.0001
..............................................................................
Attempt Newton solution of steady-state problem.
Newton steady-state solve succeeded.
Problem solved on [10] point grid(s).
..............................................................................
no new points needed in flame
Multiplier = 0.001
..............................................................................
Attempt Newton solution of steady-state problem.
Newton steady-state solve succeeded.
Problem solved on [10] point grid(s).
..............................................................................
no new points needed in flame
Multiplier = 0.01
..............................................................................
Attempt Newton solution of steady-state problem.
Newton steady-state solve succeeded.
Problem solved on [10] point grid(s).
..............................................................................
no new points needed in flame
Multiplier = 0.1
..............................................................................
Attempt Newton solution of steady-state problem.
Newton steady-state solve succeeded.
Problem solved on [10] point grid(s).
..............................................................................
no new points needed in flame
Multiplier = 1.0
..............................................................................
Attempt Newton solution of steady-state problem.
Newton steady-state solve succeeded.
Problem solved on [10] point grid(s).
..............................................................................
no new points needed in flame
At this point, we should have the solution for the hydrogen/air problem.
sim.show()
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>> inlet <<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
Mass Flux: 0.06 kg/m^2/s
Temperature: 300 K
Mass Fractions:
H2 0.003467
O2 0.2311
AR 0.01374
N2 0.7516
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>> flame <<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
Pressure: 1.013e+05 Pa
-------------------------------------------------------------------------------
z velocity spread_rate T lambda eField
-------------------------------------------------------------------------------
0 0.05335 1.988e-21 300 -0.4 0
0.02 0.05068 0.1333 300 -0.4 0
0.04 0.04268 0.2666 300 -0.4 0
0.06 0.02939 0.4001 300.6 -0.4 0
0.07 0.02105 0.4687 305.4 -0.4 0
0.08 0.013 0.5514 347.5 -0.4 0
0.085 0.009565 0.5931 426.2 -0.4 0
0.09 0.005933 0.577 569 -0.4 0
0.095 0.001988 0.3977 740.3 -0.4 0
0.1 0 0 900 -0.4 0
-------------------------------------------------------------------------------
z Uo H2 H O O2
-------------------------------------------------------------------------------
0 0 0.003467 -5.58e-21 8.646e-20 0.2311
0.02 0 0.003467 2.455e-27 2.59e-20 0.2311
0.04 0 0.003462 1.255e-27 4.737e-21 0.2311
0.06 0 0.003399 1.437e-27 1.157e-20 0.2311
0.07 0 0.003197 6.892e-26 4.789e-19 0.2308
0.08 0 0.002515 3.924e-24 1.009e-17 0.2273
0.085 0 0.001917 7.589e-22 4.992e-17 0.2199
0.09 0 0.001239 2.823e-18 1.565e-15 0.2059
0.095 0 0.000594 1.554e-14 4.715e-13 0.1886
0.1 0 1.728e-05 2.758e-17 8.599e-16 0.1721
-------------------------------------------------------------------------------
z OH H2O HO2 H2O2 C
-------------------------------------------------------------------------------
0 1.634e-24 6.854e-10 3.751e-16 4.325e-16 -4.456e-21
0.02 3.797e-23 3.18e-08 1.903e-14 2.209e-14 -6.503e-29
0.04 6.961e-24 1.404e-06 9.186e-13 1.074e-12 -8.274e-37
0.06 1.452e-23 5.255e-05 3.755e-11 4.423e-11 -6.675e-45
0.07 3.697e-22 0.0005515 4.291e-10 5.069e-10 3.331e-42
0.08 7.812e-21 0.005051 4.417e-09 5.105e-09 7.869e-34
0.085 3.041e-19 0.01333 1.441e-08 1.379e-08 -7.557e-40
0.09 2.408e-16 0.0275 3.848e-08 2.821e-08 -1.965e-34
0.095 2.228e-12 0.04362 7.898e-08 4.094e-08 -6.639e-27
0.1 1.912e-08 0.05837 7.921e-08 4.107e-08 -1.258e-19
-------------------------------------------------------------------------------
z CH CH2 CH2(S) CH3 CH4
-------------------------------------------------------------------------------
0 3.902e-20 7.533e-23 4.291e-38 1.034e-20 7.988e-22
0.02 1.775e-28 4.757e-29 1.965e-36 1.256e-20 1.011e-21
0.04 -1.581e-38 1.207e-35 4.355e-41 1.223e-20 1.331e-21
0.06 5.329e-45 -1.569e-37 1.847e-39 9.145e-21 4.379e-21
0.07 1.75e-41 -2.02e-36 2.292e-38 -7.719e-22 2.988e-20
0.08 8.912e-33 4.118e-33 9.183e-38 -4.353e-21 2.25e-19
0.085 1.093e-39 -8.583e-34 1.17e-37 -1.035e-21 4.623e-19
0.09 2.787e-34 -4.741e-32 -7.207e-37 -5.254e-23 6.727e-19
0.095 1.612e-26 -5.668e-27 -2.861e-30 3.251e-22 7.939e-19
0.1 3.881e-19 -5.802e-27 -3.023e-30 3.155e-22 9.034e-19
-------------------------------------------------------------------------------
z CO CO2 HCO CH2O CH2OH
-------------------------------------------------------------------------------
0 -1.046e-21 3.37e-24 7.103e-30 -1.07e-22 1.341e-42
0.02 -6.558e-20 2.239e-22 4.72e-28 -7.169e-21 -1.877e-41
0.04 -6.556e-20 2.175e-22 -5.823e-35 -6.988e-21 1.948e-41
0.06 -6.485e-20 -6.562e-23 -4.65e-38 -7.516e-22 9.14e-40
0.07 -5.719e-20 -1.156e-21 -6.349e-37 3.655e-20 1.272e-38
0.08 2.144e-20 8.143e-20 8.314e-32 2.723e-19 3.842e-37
0.085 1.929e-19 7.64e-19 2.015e-32 8.34e-19 2.861e-35
0.09 5.394e-19 3.235e-18 5.253e-30 2.06e-18 5.605e-32
0.095 9.837e-19 7.243e-18 2.691e-25 3.68e-18 1.801e-25
0.1 2.774e-19 1.126e-17 2.312e-25 3.733e-18 1.388e-18
-------------------------------------------------------------------------------
z CH3O CH3OH C2H C2H2 C2H3
-------------------------------------------------------------------------------
0 5.21e-30 -1.52e-21 -5.754e-20 1.114e-21 -6.468e-21
0.02 3.517e-28 -1.52e-21 -5.133e-28 9.27e-22 -6.762e-28
0.04 1.669e-26 -1.517e-21 -3.51e-36 9.267e-22 -5.969e-35
0.06 6.449e-25 -1.42e-21 -2.447e-44 9.1e-22 -7.474e-42
0.07 5.001e-24 -1.014e-21 -6.908e-49 6.922e-22 -1.507e-40
0.08 1.929e-24 -7.768e-22 -1.049e-45 -1.89e-21 -1.322e-37
0.085 -7.659e-25 -1.691e-21 -1.936e-42 -7.935e-21 -4.398e-35
0.09 -4.555e-27 -2.927e-21 5.321e-34 -2.047e-20 -1.71e-32
0.095 2.847e-25 -4.566e-21 1.688e-26 -3.682e-20 1.374e-28
0.1 2.805e-25 -6.378e-21 1.455e-19 -5.126e-20 1.41e-28
-------------------------------------------------------------------------------
z C2H4 C2H5 C2H6 HCCO CH2CO
-------------------------------------------------------------------------------
0 6.387e-21 -1.07e-40 -6.295e-21 9.022e-41 2.249e-21
0.02 6.387e-21 -7.198e-39 -6.295e-21 3.859e-39 2.249e-21
0.04 6.381e-21 2.342e-38 -6.295e-21 -8.334e-39 2.249e-21
0.06 6.078e-21 8.867e-37 -6.295e-21 -3.721e-37 2.248e-21
0.07 1.956e-21 1.027e-35 -6.296e-21 -5.597e-36 2.238e-21
0.08 -4.943e-20 1.761e-34 -6.325e-21 -2.562e-34 2.087e-21
0.085 -1.773e-19 -2.323e-33 -6.398e-21 -8.228e-33 1.65e-21
0.09 -4.567e-19 -2.066e-28 -6.568e-21 -5.955e-31 5.872e-22
0.095 -8.311e-19 -8.746e-24 -6.809e-21 -9.412e-29 -9.211e-22
0.1 -8.338e-19 -4.064e-19 -7.141e-21 -8.084e-29 -2.399e-21
-------------------------------------------------------------------------------
z HCCOH AR N2
-------------------------------------------------------------------------------
0 3.585e-21 0.01374 0.7516
0.02 3.585e-21 0.01374 0.7516
0.04 3.585e-21 0.01374 0.7516
0.06 3.585e-21 0.01374 0.7517
0.07 3.591e-21 0.01374 0.7517
0.08 3.679e-21 0.01374 0.7514
0.085 3.946e-21 0.01373 0.7511
0.09 4.622e-21 0.01374 0.7516
0.095 5.602e-21 0.01378 0.7534
0.1 6.566e-21 0.01382 0.7557
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>> surface <<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
Temperature: 900 K
Coverages:
PT(S) 0.08832
H(S) 1.352e-07
H2O(S) 1.677e-05
OH(S) 0.00567
CO(S) -6.891e-21
CO2(S) 2.283e-26
CH3(S) 8.052e-24
CH2(S)s 8.052e-24
CH(S) 8.052e-24
C(S) -2.944e-23
O(S) 0.906
Now switch the inlet to the methane/air composition.
sim.inlet.X = comp2
# set more stringent grid refinement criteria
sim.set_refine_criteria(100.0, 0.15, 0.2, 0.0)
# solve the problem for the final time
sim.solve(loglevel)
..............................................................................
Attempt Newton solution of steady-state problem.
Newton steady-state solve succeeded.
Problem solved on [10] point grid(s).
..............................................................................
##############################################################################
Refining grid in flame.
New points inserted after grid points 0 1 2 3 4 5 6 7 8
to resolve AR C2H6 CH2O CH4 CO CO2 H2O N2 O2 OH T spread_rate velocity
##############################################################################
..............................................................................
Attempt Newton solution of steady-state problem.
Newton steady-state solve succeeded.
Problem solved on [19] point grid(s).
..............................................................................
##############################################################################
Refining grid in flame.
New points inserted after grid points 9 10 11 12 13 14 15 16 17
to resolve AR CH2O CH4 CO CO2 H2O N2 O2 OH T spread_rate velocity
##############################################################################
..............................................................................
Attempt Newton solution of steady-state problem.
Newton steady-state solve succeeded.
Problem solved on [28] point grid(s).
..............................................................................
##############################################################################
Refining grid in flame.
New points inserted after grid points 24 25 26
to resolve OH spread_rate velocity
##############################################################################
..............................................................................
Attempt Newton solution of steady-state problem.
Newton steady-state solve succeeded.
Problem solved on [31] point grid(s).
..............................................................................
##############################################################################
Refining grid in flame.
New points inserted after grid points 29
to resolve OH
##############################################################################
..............................................................................
Attempt Newton solution of steady-state problem.
Newton steady-state solve succeeded.
Problem solved on [32] point grid(s).
..............................................................................
no new points needed in flame
show the solution
sim.show()
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>> inlet <<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
Mass Flux: 0.06 kg/m^2/s
Temperature: 300 K
Mass Fractions:
O2 0.2204
CH4 0.04998
AR 0.0131
N2 0.7166
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>> flame <<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
Pressure: 1.013e+05 Pa
-------------------------------------------------------------------------------
z velocity spread_rate T lambda eField
-------------------------------------------------------------------------------
0 0.05304 5.908e-25 300 -0.3867 0
0.01 0.05239 0.06444 300 -0.3867 0
0.02 0.05046 0.1289 300 -0.3867 0
0.03 0.04724 0.1933 300 -0.3867 0
0.04 0.04273 0.2578 300 -0.3867 0
0.05 0.03693 0.3222 300 -0.3867 0
0.06 0.02984 0.3867 300 -0.3867 0
0.065 0.02582 0.4189 300.1 -0.3867 0
0.07 0.02149 0.4513 300.4 -0.3867 0
0.075 0.01693 0.4842 302.2 -0.3867 0
0.0775 0.01465 0.5017 305.6 -0.3867 0
0.08 0.01247 0.521 314.3 -0.3867 0
0.08125 0.01147 0.5318 322.7 -0.3867 0
0.0825 0.01056 0.5434 335.5 -0.3867 0
0.08375 0.009736 0.5556 354 -0.3867 0
0.085 0.008971 0.5673 378.9 -0.3867 0
0.08625 0.008228 0.5766 410.4 -0.3867 0
0.0875 0.007466 0.5813 447.9 -0.3867 0
0.08875 0.006649 0.5789 490.3 -0.3867 0
0.09 0.005765 0.5668 536 -0.3867 0
0.09125 0.004822 0.5433 583.7 -0.3867 0
0.0925 0.003848 0.5069 632.1 -0.3867 0
0.09375 0.002883 0.4567 680.2 -0.3867 0
0.095 0.001979 0.3925 727.3 -0.3867 0
0.09625 0.001186 0.3144 773 -0.3867 0
0.09688 0.0008485 0.2702 795.2 -0.3867 0
0.0975 0.0005586 0.2226 817 -0.3867 0
0.09813 0.0003229 0.1717 838.4 -0.3867 0
0.09875 0.0001473 0.1176 859.4 -0.3867 0
0.09938 3.774e-05 0.06034 879.9 -0.3867 0
0.09969 9.583e-06 0.03055 890 -0.3867 0
0.1 0 0 900 -0.3867 0
-------------------------------------------------------------------------------
z Uo H2 H O O2
-------------------------------------------------------------------------------
0 0 -5.538e-18 6.48e-23 1.188e-19 0.2204
0.01 0 -4.315e-17 2.055e-28 5.991e-20 0.2204
0.02 0 -3.332e-16 2.862e-34 2.045e-20 0.2204
0.03 0 -2.497e-15 2.159e-31 6.695e-21 0.2204
0.04 0 -1.775e-14 -5.727e-32 2.055e-21 0.2204
0.05 0 -1.165e-13 5.303e-33 5.732e-22 0.2204
0.06 0 -6.821e-13 7.803e-32 1.467e-22 0.2204
0.065 0 -1.775e-12 2.161e-31 1.827e-22 0.2203
0.07 0 -4.674e-12 4.82e-29 1.793e-21 0.2203
0.075 0 -1.154e-11 1.212e-26 2.311e-20 0.22
0.0775 0 -1.77e-11 2.161e-25 1.243e-19 0.2194
0.08 0 -2.654e-11 3.667e-24 5.612e-19 0.2177
0.08125 0 -3.209e-11 1.466e-23 1.152e-18 0.216
0.0825 0 -3.841e-11 5.539e-23 2.097e-18 0.2132
0.08375 0 -4.539e-11 1.904e-22 3.184e-18 0.209
0.085 0 -5.287e-11 6.075e-22 3.869e-18 0.2032
0.08625 0 -6.068e-11 2.312e-21 3.859e-18 0.1957
0.0875 0 -6.861e-11 1.987e-20 3.72e-18 0.1864
0.08875 0 -7.652e-11 2.864e-19 6.284e-18 0.1757
0.09 0 -8.428e-11 3.575e-18 3.116e-17 0.1638
0.09125 0 -9.182e-11 3.271e-17 2.062e-16 0.1512
0.0925 0 -9.91e-11 2.154e-16 1.207e-15 0.1382
0.09375 0 -1.061e-10 1.033e-15 5.892e-15 0.1251
0.095 0 -1.129e-10 3.759e-15 2.403e-14 0.1122
0.09625 0 -1.198e-10 1.124e-14 8.456e-14 0.09946
0.09688 0 -1.238e-10 1.876e-14 1.54e-13 0.09326
0.0975 0 -1.283e-10 3.082e-14 2.753e-13 0.08715
0.09813 0 -1.34e-10 5.036e-14 4.869e-13 0.08115
0.09875 0 -1.417e-10 8.257e-14 8.591e-13 0.07525
0.09938 0 -1.53e-10 1.371e-13 1.524e-12 0.06945
0.09969 0 -1.611e-10 1.809e-13 2.006e-12 0.06659
0.1 0 -1.71e-10 3.422e-15 3.9e-14 0.06376
-------------------------------------------------------------------------------
z OH H2O HO2 H2O2 C
-------------------------------------------------------------------------------
0 2.143e-24 1.356e-14 6.981e-21 -4.667e-21 3.937e-20
0.01 3.766e-23 3.307e-13 1.847e-19 8.74e-22 1.27e-27
0.02 1.277e-23 7.976e-12 4.879e-19 1.464e-19 3.955e-35
0.03 4.181e-24 1.858e-10 8.048e-18 3.885e-18 1.158e-42
0.04 1.286e-24 4.068e-09 1.869e-16 9.302e-17 3.084e-50
0.05 1.491e-24 8.112e-08 4.031e-15 2.023e-15 7.176e-58
0.06 3.703e-22 1.413e-06 7.596e-14 3.84e-14 1.417e-65
0.065 1.493e-20 8.652e-06 4.98e-13 2.534e-13 1.454e-68
0.07 6.964e-19 5.707e-05 3.531e-12 1.808e-12 -1.293e-65
0.075 2.467e-17 0.0003335 2.212e-11 1.14e-11 -5.67e-64
0.0775 1.588e-16 0.0008499 5.923e-11 3.061e-11 -2.126e-61
0.08 9.223e-16 0.002149 1.584e-10 8.2e-11 5.977e-60
0.08125 2.076e-15 0.003384 2.58e-10 1.335e-10 2.827e-59
0.0825 4.287e-15 0.005243 4.153e-10 2.143e-10 -9.718e-58
0.08375 7.865e-15 0.007865 6.487e-10 3.331e-10 8.937e-57
0.085 1.28e-14 0.01132 9.735e-10 4.964e-10 2.523e-56
0.08625 1.888e-14 0.01558 1.398e-09 7.06e-10 -8.255e-53
0.0875 2.616e-14 0.02052 1.921e-09 9.587e-10 1.725e-49
0.08875 3.535e-14 0.02596 2.534e-09 1.247e-09 3.263e-43
0.09 4.856e-14 0.03173 3.223e-09 1.561e-09 2.362e-37
0.09125 7.209e-14 0.03764 3.972e-09 1.89e-09 -2.374e-38
0.0925 1.256e-13 0.04356 4.768e-09 2.224e-09 -2.67e-37
0.09375 2.696e-13 0.04939 5.604e-09 2.555e-09 -2.189e-31
0.095 6.824e-13 0.05507 6.475e-09 2.87e-09 8.811e-36
0.09625 1.882e-12 0.06056 7.385e-09 3.149e-09 5.482e-30
0.09688 3.208e-12 0.06322 7.852e-09 3.263e-09 -9.86e-35
0.0975 5.507e-12 0.06584 8.322e-09 3.354e-09 -1.784e-29
0.09813 9.543e-12 0.0684 8.782e-09 3.417e-09 -4.325e-28
0.09875 1.677e-11 0.07091 9.206e-09 3.449e-09 1.306e-27
0.09938 3.07e-11 0.07337 9.539e-09 3.455e-09 -1.235e-28
0.09969 1.934e-10 0.07458 9.63e-09 3.453e-09 -4.396e-24
0.1 1.749e-08 0.07579 9.628e-09 3.452e-09 -8.476e-22
-------------------------------------------------------------------------------
z CH CH2 CH2(S) CH3 CH4
-------------------------------------------------------------------------------
0 4.425e-35 1.91e-21 2.098e-36 3.711e-20 0.04998
0.01 3.373e-43 1.258e-27 5.031e-35 2.627e-19 0.04998
0.02 8.633e-48 7.992e-34 3.011e-41 6.439e-19 0.04998
0.03 1.371e-48 6.196e-39 2.775e-40 7.768e-18 0.04998
0.04 1.027e-46 5.386e-38 2.556e-39 1.636e-16 0.04998
0.05 -4.105e-46 9.511e-37 4.548e-38 3.271e-15 0.04998
0.06 3.504e-44 1.188e-33 6.166e-35 5.726e-14 0.04998
0.065 -3.493e-43 2.919e-31 1.517e-32 3.521e-13 0.04998
0.07 -2.065e-41 9.019e-29 4.69e-30 2.333e-12 0.04995
0.075 -4.059e-42 1.878e-26 9.808e-28 1.373e-11 0.04984
0.0775 -2.164e-40 3.117e-25 1.641e-26 3.544e-11 0.04962
0.08 4.482e-40 4.671e-24 2.503e-25 9.187e-11 0.04909
0.08125 1.207e-39 1.698e-23 9.245e-25 1.492e-10 0.04858
0.0825 -1.964e-38 5.62e-23 3.127e-24 2.414e-10 0.04781
0.08375 4.115e-38 1.619e-22 9.249e-24 3.852e-10 0.04672
0.085 7.229e-38 4.034e-22 2.367e-23 6.033e-10 0.04524
0.08625 -1.02e-38 8.907e-22 5.345e-23 9.276e-10 0.04339
0.0875 -3.871e-37 1.819e-21 1.104e-22 1.403e-09 0.04118
0.08875 2.378e-36 3.606e-21 2.181e-22 2.096e-09 0.03868
0.09 2.049e-35 7.335e-21 4.338e-22 3.104e-09 0.03596
0.09125 2.913e-34 1.652e-20 9.355e-22 4.575e-09 0.03309
0.0925 5.004e-33 4.51e-20 2.394e-21 6.748e-09 0.03016
0.09375 8.69e-32 1.548e-19 7.586e-21 1.002e-08 0.02722
0.095 1.362e-30 6.331e-19 2.839e-20 1.511e-08 0.02431
0.09625 2.047e-29 2.883e-18 1.175e-19 2.335e-08 0.02146
0.09688 8.137e-29 6.467e-18 2.506e-19 2.969e-08 0.02006
0.0975 3.265e-28 1.48e-17 5.44e-19 3.815e-08 0.01869
0.09813 1.347e-27 3.477e-17 1.211e-18 4.972e-08 0.01734
0.09875 5.817e-27 8.472e-17 2.787e-18 6.606e-08 0.016
0.09938 1.738e-26 2.242e-16 6.944e-18 9e-08 0.01469
0.09969 -1.275e-23 2.83e-15 8.769e-17 1.066e-07 0.01405
0.1 -1.275e-23 2.829e-15 8.767e-17 1.066e-07 0.0134
-------------------------------------------------------------------------------
z CO CO2 HCO CH2O CH2OH
-------------------------------------------------------------------------------
0 3.837e-19 2.25e-16 4.059e-28 4.693e-21 -2.791e-43
0.01 1.017e-17 7.764e-15 1.405e-26 1.638e-19 -9.808e-42
0.02 2.592e-16 2.648e-13 3.307e-33 2.679e-18 8.643e-43
0.03 6.553e-15 8.717e-12 1.001e-37 8.629e-17 2.681e-41
0.04 1.559e-13 2.694e-10 1.916e-36 2.694e-15 2.99e-40
0.05 3.373e-12 7.566e-09 5.63e-34 7.651e-14 2.44e-38
0.06 6.374e-11 1.851e-07 2.459e-31 1.892e-12 1.417e-35
0.065 4.188e-10 1.529e-06 1.421e-29 1.578e-11 2.055e-33
0.07 2.976e-09 1.382e-05 1.546e-27 1.442e-10 7.012e-31
0.075 1.869e-08 0.0001091 2.607e-25 1.149e-09 1.925e-28
0.0775 5.007e-08 0.000344 4.908e-24 3.647e-09 3.933e-27
0.08 1.339e-07 0.001094 8.891e-23 1.166e-08 7.286e-26
0.08125 2.18e-07 0.001963 3.611e-22 2.098e-08 2.944e-25
0.0825 3.502e-07 0.003486 1.351e-21 3.729e-08 1.08e-24
0.08375 5.455e-07 0.005963 4.445e-21 6.375e-08 3.415e-24
0.085 8.154e-07 0.009689 1.303e-20 1.033e-07 9.29e-24
0.08625 1.165e-06 0.01487 3.661e-20 1.578e-07 2.284e-23
0.0875 1.589e-06 0.02156 1.095e-19 2.274e-07 5.671e-23
0.08875 2.079e-06 0.02965 3.677e-19 3.105e-07 1.68e-22
0.09 2.619e-06 0.03891 1.322e-18 4.042e-07 6.525e-22
0.09125 3.191e-06 0.04904 4.723e-18 5.05e-07 2.982e-21
0.0925 3.781e-06 0.05971 1.61e-17 6.094e-07 1.361e-20
0.09375 4.377e-06 0.07065 5.212e-17 7.144e-07 5.671e-20
0.095 4.967e-06 0.08163 1.625e-16 8.169e-07 2.098e-19
0.09625 5.545e-06 0.09247 4.954e-16 9.137e-07 6.981e-19
0.09688 5.828e-06 0.0978 8.67e-16 9.582e-07 1.243e-18
0.0975 6.107e-06 0.1031 1.511e-15 9.991e-07 2.194e-18
0.09813 6.381e-06 0.1082 2.629e-15 1.035e-06 3.878e-18
0.09875 6.651e-06 0.1133 4.575e-15 1.064e-06 6.977e-18
0.09938 6.916e-06 0.1184 7.962e-15 1.084e-06 1.278e-17
0.09969 7.047e-06 0.1208 7.25e-15 1.088e-06 -3.031e-17
0.1 7.177e-06 0.1233 7.249e-15 1.088e-06 -3.03e-17
-------------------------------------------------------------------------------
z CH3O CH3OH C2H C2H2 C2H3
-------------------------------------------------------------------------------
0 -1.787e-33 -1.293e-21 4.901e-20 -1.362e-21 7.743e-20
0.01 -6.279e-32 -1.016e-21 9.589e-28 -7.535e-22 1.771e-26
0.02 9.657e-32 8.701e-21 1.809e-35 -7.556e-22 3.908e-33
0.03 2.015e-29 3.375e-19 3.202e-43 -8.221e-22 8.088e-40
0.04 9.895e-27 1.077e-17 5.145e-51 -2.765e-21 4.251e-41
0.05 4.263e-24 3.114e-16 -3.06e-54 -5.433e-20 2.317e-38
0.06 1.401e-21 7.836e-15 -3.044e-51 -1.244e-18 9.854e-36
0.065 5.655e-20 6.638e-14 -9.347e-49 -9.792e-18 5.204e-34
0.07 2.652e-18 6.163e-13 -3.786e-46 -8.423e-17 4.212e-32
0.075 9.678e-17 4.99e-12 -1.164e-43 -6.342e-16 5.119e-30
0.0775 6.582e-16 1.602e-11 -3.017e-42 -1.932e-15 9.259e-29
0.08 4.362e-15 5.189e-11 -1.096e-40 -5.921e-15 1.881e-27
0.08125 1.103e-14 9.402e-11 -8.346e-40 -1.04e-14 8.813e-27
0.0825 2.673e-14 1.685e-10 -7.72e-39 -1.807e-14 4.123e-26
0.08375 5.961e-14 2.903e-10 -8.299e-38 -3.029e-14 1.86e-25
0.085 1.196e-13 4.738e-10 -9.724e-37 -4.83e-14 8.187e-25
0.08625 2.143e-13 7.287e-10 -1.14e-35 -7.294e-14 3.7e-24
0.0875 3.46e-13 1.057e-09 -1.233e-34 -1.043e-13 1.798e-23
0.08875 5.124e-13 1.451e-09 -1.194e-33 -1.418e-13 9.244e-23
0.09 7.108e-13 1.898e-09 -1.132e-32 -1.842e-13 4.695e-22
0.09125 9.346e-13 2.381e-09 -1.244e-31 -2.302e-13 2.221e-21
0.0925 1.159e-12 2.882e-09 -1.528e-30 -2.784e-13 9.562e-21
0.09375 1.34e-12 3.382e-09 -1.719e-29 -3.275e-13 3.755e-20
0.095 1.455e-12 3.862e-09 -1.609e-28 -3.766e-13 1.369e-19
0.09625 1.555e-12 4.303e-09 -1.256e-27 -4.252e-13 4.752e-19
0.09688 1.645e-12 4.502e-09 -3.333e-27 -4.493e-13 8.852e-19
0.0975 1.771e-12 4.684e-09 -8.597e-27 -4.735e-13 1.645e-18
0.09813 1.953e-12 4.845e-09 -2.16e-26 -4.978e-13 3.069e-18
0.09875 2.218e-12 4.981e-09 -5.386e-26 -5.228e-13 5.781e-18
0.09938 2.606e-12 5.085e-09 -1.015e-26 -5.49e-13 9.995e-18
0.09969 2.904e-12 5.12e-09 -4.727e-23 -5.63e-13 -2.215e-16
0.1 2.904e-12 5.119e-09 4.517e-21 -5.629e-13 -2.214e-16
-------------------------------------------------------------------------------
z C2H4 C2H5 C2H6 HCCO CH2CO
-------------------------------------------------------------------------------
0 1.388e-21 -9.664e-40 1.276e-19 -1.228e-41 -2.892e-28
0.01 1.986e-21 -3.524e-38 1.725e-19 -2.692e-40 -1.657e-28
0.02 2.203e-20 -9.837e-33 1.803e-18 -9.189e-41 4.754e-27
0.03 6.698e-19 2.567e-32 5.898e-17 -3.016e-41 1.937e-25
0.04 2.031e-17 3.143e-31 1.937e-15 -1.238e-41 6.996e-24
0.05 5.607e-16 5.108e-30 5.792e-14 -8.565e-41 2.292e-22
0.06 1.349e-14 9.051e-29 1.508e-12 -4.633e-40 6.533e-21
0.065 1.097e-13 1.128e-27 1.317e-11 3.899e-39 6.195e-20
0.07 9.761e-13 1.166e-25 1.262e-10 2.385e-36 6.471e-19
0.075 7.589e-12 2.653e-23 1.054e-09 7.639e-34 5.866e-18
0.0775 2.366e-11 5.323e-22 3.464e-09 1.757e-32 2.05e-17
0.08 7.432e-11 9.501e-21 1.151e-08 3.844e-31 7.272e-17
0.08125 1.324e-10 3.704e-20 2.119e-08 1.752e-30 1.391e-16
0.0825 2.332e-10 1.287e-19 3.865e-08 7.462e-30 2.638e-16
0.08375 3.958e-10 3.772e-19 6.779e-08 2.786e-29 4.797e-16
0.085 6.381e-10 9.368e-19 1.126e-07 8.936e-29 8.222e-16
0.08625 9.721e-10 2.089e-18 1.761e-07 2.485e-28 1.32e-15
0.0875 1.4e-09 4.652e-18 2.593e-07 6.156e-28 1.987e-15
0.08875 1.913e-09 1.166e-17 3.611e-07 1.402e-27 2.815e-15
0.09 2.496e-09 3.432e-17 4.783e-07 3.025e-27 3.782e-15
0.09125 3.129e-09 1.112e-16 6.066e-07 6.251e-27 4.854e-15
0.0925 3.792e-09 3.67e-16 7.415e-07 1.163e-26 5.993e-15
0.09375 4.466e-09 1.19e-15 8.785e-07 1.267e-26 7.166e-15
0.095 5.138e-09 3.78e-15 1.013e-06 -4.391e-26 8.344e-15
0.09625 5.791e-09 1.199e-14 1.141e-06 -4.384e-25 9.506e-15
0.09688 6.104e-09 2.18e-14 1.201e-06 -1.068e-24 1.007e-14
0.0975 6.404e-09 4.005e-14 1.256e-06 -2.446e-24 1.063e-14
0.09813 6.686e-09 7.498e-14 1.305e-06 -5.349e-24 1.117e-14
0.09875 6.938e-09 1.443e-13 1.347e-06 -1.148e-23 1.168e-14
0.09938 7.144e-09 2.973e-13 1.375e-06 -4.1e-23 1.215e-14
0.09969 7.216e-09 1.105e-12 1.381e-06 -3.455e-21 1.234e-14
0.1 7.214e-09 1.105e-12 1.381e-06 -3.454e-21 1.234e-14
-------------------------------------------------------------------------------
z HCCOH AR N2
-------------------------------------------------------------------------------
0 8.222e-32 0.0131 0.7166
0.01 -1.053e-32 0.0131 0.7166
0.02 -3.705e-30 0.0131 0.7166
0.03 -1.456e-28 0.0131 0.7166
0.04 -5.253e-27 0.0131 0.7166
0.05 -1.721e-25 0.0131 0.7166
0.06 -4.905e-24 0.0131 0.7166
0.065 -4.652e-23 0.0131 0.7166
0.07 -4.859e-22 0.0131 0.7166
0.075 -4.405e-21 0.0131 0.7166
0.0775 -1.539e-20 0.0131 0.7167
0.08 -5.46e-20 0.01311 0.7169
0.08125 -1.044e-19 0.01311 0.717
0.0825 -1.981e-19 0.01311 0.7172
0.08375 -3.602e-19 0.01311 0.7173
0.085 -6.174e-19 0.01312 0.7174
0.08625 -9.913e-19 0.01312 0.7174
0.0875 -1.492e-18 0.01311 0.7172
0.08875 -2.114e-18 0.01311 0.7169
0.09 -2.84e-18 0.0131 0.7165
0.09125 -3.644e-18 0.01309 0.7159
0.0925 -4.5e-18 0.01308 0.7153
0.09375 -5.381e-18 0.01306 0.7145
0.095 -6.266e-18 0.01305 0.7138
0.09625 -7.139e-18 0.01304 0.713
0.09688 -7.568e-18 0.01303 0.7126
0.0975 -7.99e-18 0.01302 0.7122
0.09813 -8.405e-18 0.01302 0.7119
0.09875 -8.812e-18 0.01301 0.7115
0.09938 -9.212e-18 0.013 0.7111
0.09969 -9.41e-18 0.013 0.7109
0.1 -9.408e-18 0.013 0.7108
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>> surface <<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<
Temperature: 900 K
Coverages:
PT(S) 0.1251
H(S) 2.668e-07
H2O(S) 3.12e-05
OH(S) 0.007566
CO(S) 3.863e-05
CO2(S) 4.183e-10
CH3(S) 3.124e-09
CH2(S)s 3.124e-09
CH(S) 3.124e-09
C(S) 1.373e-07
O(S) 0.8673
Save the full solution to HDF or YAML container files. The restore
method can be
used to restore or restart a simulation from a solution stored in this form.
if "native" in ct.hdf_support():
filename = "catalytic_combustion.h5"
else:
filename = "catalytic_combustion.yaml"
sim.save(filename, "soln1", description="catalytic combustion example",
overwrite=True)
# save selected solution components in a CSV file for plotting in Excel or MATLAB.
sim.save('catalytic_combustion.csv', basis="mole", overwrite=True)
sim.show_stats(0)
Statistics:
Grid Timesteps Functions Time Jacobians Time
8 0 84 NA 1 NA
10 0 6 NA 1 NA
12 0 6 NA 1 NA
12 0 12 NA 1 NA
12 0 6 NA 1 NA
12 0 10 NA 1 NA
12 0 18 NA 1 NA
12 0 14 NA 1 NA
12 0 6 NA 1 NA
12 0 66 NA 3 NA
21 0 32 NA 2 NA
30 0 32 NA 2 NA
33 0 23 NA 1 NA
34 0 19 NA 1 NA
Temperature Profile#
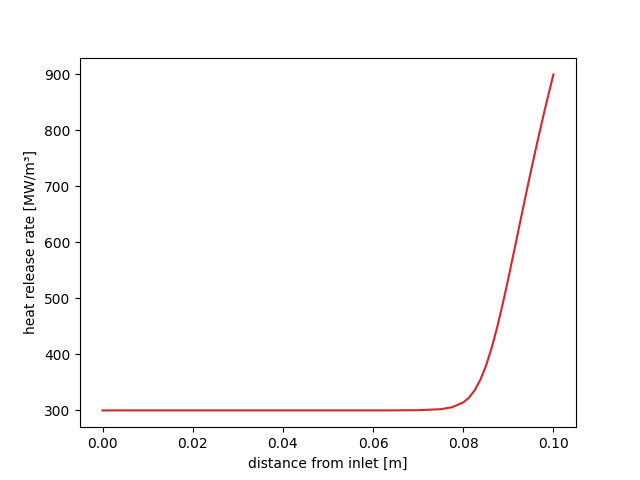
Major Species Profiles#
fig, ax = plt.subplots()
major = ('O2', 'CH4', 'H2O', 'CO2')
states = sim.to_array()
ax.plot(states.grid, states(*major).X, label=major)
ax.set(xlabel='distance from inlet [m]', ylabel='mole fractions')
ax.legend()
plt.show()
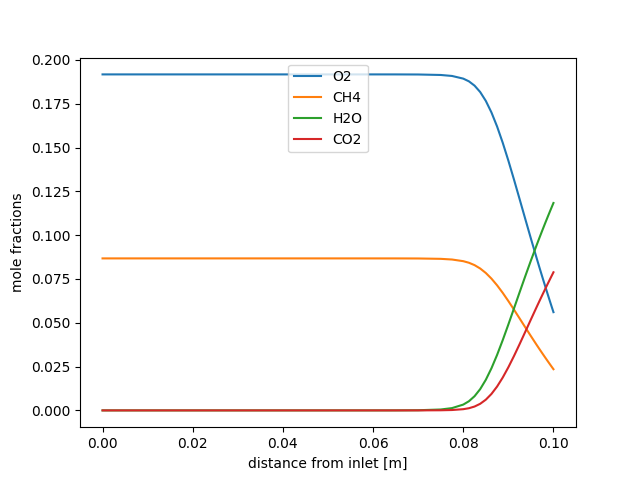
Total running time of the script: (0 minutes 1.006 seconds)