Note
Go to the end to download the full example code.
Diffusion flame extinction strain rate#
This example computes the extinction point of a counterflow diffusion flame. A hydrogen-oxygen diffusion flame at 1 bar is studied.
The tutorial makes use of the scaling rules derived by Fiala and Sattelmayer (doi:10.1155/2014/484372). Please refer to this publication for a detailed explanation. Also, please don’t forget to cite it if you make use of it.
Requires: cantera >= 3.0, matplotlib >= 2.0
from pathlib import Path
import numpy as np
import matplotlib.pyplot as plt
import cantera as ct
Initialization#
Set up an initial hydrogen-oxygen counterflow flame at 1 bar and low strain rate (maximum axial velocity gradient = 2414 1/s)
reaction_mechanism = 'h2o2.yaml'
gas = ct.Solution(reaction_mechanism)
width = 18.e-3 # 18mm wide
f = ct.CounterflowDiffusionFlame(gas, width=width)
# Define the operating pressure and boundary conditions
f.P = 1.e5 # 1 bar
f.fuel_inlet.mdot = 0.5 # kg/m^2/s
f.fuel_inlet.X = 'H2:1'
f.fuel_inlet.T = 300 # K
f.oxidizer_inlet.mdot = 3.0 # kg/m^2/s
f.oxidizer_inlet.X = 'O2:1'
f.oxidizer_inlet.T = 500 # K
# Set refinement parameters
f.set_refine_criteria(ratio=3.0, slope=0.1, curve=0.2, prune=0.03)
# Define a limit for the maximum temperature below which the flame is
# considered as extinguished and the computation is aborted
temperature_limit_extinction = max(f.oxidizer_inlet.T, f.fuel_inlet.T)
# Initialize and solve
print('Creating the initial solution')
f.solve(loglevel=0, auto=True)
# Define output locations
output_path = Path() / "diffusion_flame_extinction_data"
output_path.mkdir(parents=True, exist_ok=True)
hdf_output = "native" in ct.hdf_support()
if hdf_output:
file_name = output_path / "flame_data.h5"
file_name.unlink(missing_ok=True)
def names(test):
if hdf_output:
# use internal container structure for HDF
file_name = output_path / "flame_data.h5"
return file_name, test
# use separate files for YAML
file_name = output_path / f"{test}.yaml".replace("-", "_").replace("/", "_")
return file_name, "solution"
file_name, entry = names("initial-solution")
f.save(file_name, name=entry, description="Initial solution", overwrite=True)
Creating the initial solution
Compute Extinction Strain Rate#
Exponents for the initial solution variation with changes in strain rate Taken from Fiala and Sattelmayer (2014)
exp_d_a = - 1. / 2.
exp_u_a = 1. / 2.
exp_V_a = 1.
exp_lam_a = 2.
exp_mdot_a = 1. / 2.
# Set normalized initial strain rate
alpha = [1.]
# Initial relative strain rate increase
delta_alpha = 1.
# Factor of refinement of the strain rate increase
delta_alpha_factor = 50.
# Limit of the refinement: Minimum normalized strain rate increase
delta_alpha_min = .001
# Limit of the Temperature decrease
delta_T_min = 1 # K
# Iteration indicator
n = 0
# Indicator of the latest flame still burning
n_last_burning = 0
# List of peak temperatures
T_max = [np.max(f.T)]
# List of maximum axial velocity gradients
a_max = [np.max(np.abs(np.gradient(f.velocity) / np.gradient(f.grid)))]
Simulate counterflow flames at increasing strain rates until the flame is extinguished. To achieve a fast simulation, an initial coarse strain rate increase is set. This increase is reduced after an extinction event and the simulation is again started based on the last burning solution. The extinction point is considered to be reached if the abortion criteria on strain rate increase and peak temperature decrease are fulfilled.
while True:
n += 1
# Update relative strain rates
alpha.append(alpha[n_last_burning] + delta_alpha)
strain_factor = alpha[-1] / alpha[n_last_burning]
# Create an initial guess based on the previous solution
# Update grid
# Note that grid scaling changes the diffusion flame width
f.flame.grid *= strain_factor ** exp_d_a
normalized_grid = f.grid / (f.grid[-1] - f.grid[0])
# Update mass fluxes
f.fuel_inlet.mdot *= strain_factor ** exp_mdot_a
f.oxidizer_inlet.mdot *= strain_factor ** exp_mdot_a
# Update velocities
f.set_profile('velocity', normalized_grid,
f.velocity * strain_factor ** exp_u_a)
f.set_profile('spread_rate', normalized_grid,
f.spread_rate * strain_factor ** exp_V_a)
# Update pressure curvature
f.set_profile('lambda', normalized_grid, f.L * strain_factor ** exp_lam_a)
try:
f.solve(loglevel=0)
except ct.CanteraError as e:
print('Error: Did not converge at n =', n, e)
T_max.append(np.max(f.T))
a_max.append(np.max(np.abs(np.gradient(f.velocity) / np.gradient(f.grid))))
if not np.isclose(np.max(f.T), temperature_limit_extinction):
# Flame is still burning, so proceed to next strain rate
n_last_burning = n
file_name, entry = names(f"extinction/{n:04d}")
f.save(file_name, name=entry, description=f"Solution at alpha = {alpha[-1]}",
overwrite=True)
print('Flame burning at alpha = {:8.4F}. Proceeding to the next iteration, '
'with delta_alpha = {}'.format(alpha[-1], delta_alpha))
elif ((T_max[-2] - T_max[-1] < delta_T_min) and (delta_alpha < delta_alpha_min)):
# If the temperature difference is too small and the minimum relative
# strain rate increase is reached, save the last, non-burning, solution
# to the output file and break the loop
file_name, entry = names(f"extinction/{n:04d}")
f.save(file_name, name=entry, overwrite=True,
description=f"Flame extinguished at alpha={alpha[-1]}")
print('Flame extinguished at alpha = {0:8.4F}.'.format(alpha[-1]),
'Abortion criterion satisfied.')
break
else:
# Procedure if flame extinguished but abortion criterion is not satisfied
# Reduce relative strain rate increase
delta_alpha = delta_alpha / delta_alpha_factor
print('Flame extinguished at alpha = {0:8.4F}. Restoring alpha = {1:8.4F} and '
'trying delta_alpha = {2}'.format(
alpha[-1], alpha[n_last_burning], delta_alpha))
# Restore last burning solution
file_name, entry = names(f"extinction/{n_last_burning:04d}")
f.restore(file_name, entry)
Flame burning at alpha = 2.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 3.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 4.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 5.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 6.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 7.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 8.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 9.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 10.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 11.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 12.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 13.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 14.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 15.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 16.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 17.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 18.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 19.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 20.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 21.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 22.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 23.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 24.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 25.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 26.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 27.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 28.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 29.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 30.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 31.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 32.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 33.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 34.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 35.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 36.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 37.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 38.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 39.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 40.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 41.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 42.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 43.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 44.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 45.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 46.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 47.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 48.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 49.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 50.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 51.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 52.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 53.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 54.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 55.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 56.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 57.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 58.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 59.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 60.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 61.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 62.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 63.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 64.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 65.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 66.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 67.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 68.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 69.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 70.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 71.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 72.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 73.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 74.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 75.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 76.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 77.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 78.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 79.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 80.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 81.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 82.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 83.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 84.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 85.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 86.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 87.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 88.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 89.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 90.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 91.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 92.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 93.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 94.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 95.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 96.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 97.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 98.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 99.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 100.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 101.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 102.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 103.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 104.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 105.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 106.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 107.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 108.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 109.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 110.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 111.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 112.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 113.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 114.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 115.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 116.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 117.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 118.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 119.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 120.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 121.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 122.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 123.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 124.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 125.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 126.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 127.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 128.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 129.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 130.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 131.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 132.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 133.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 134.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 135.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 136.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 137.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 138.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 139.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 140.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 141.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 142.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 143.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 144.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 145.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 146.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 147.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 148.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 149.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 150.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 151.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 152.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 153.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 154.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 155.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 156.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 157.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 158.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 159.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 160.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 161.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 162.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 163.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 164.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 165.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 166.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 167.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 168.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 169.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 170.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 171.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 172.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 173.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 174.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 175.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 176.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 177.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 178.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 179.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 180.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 181.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 182.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 183.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 184.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 185.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 186.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 187.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 188.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 189.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 190.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 191.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 192.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 193.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 194.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 195.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 196.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 197.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 198.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 199.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 200.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 201.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 202.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 203.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 204.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 205.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 206.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 207.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 208.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 209.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 210.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 211.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 212.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 213.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 214.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 215.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 216.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 217.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 218.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 219.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 220.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 221.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 222.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 223.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 224.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 225.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 226.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 227.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 228.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 229.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 230.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 231.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 232.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 233.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 234.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 235.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 236.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 237.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 238.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 239.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 240.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 241.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 242.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 243.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 244.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 245.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 246.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 247.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 248.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 249.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 250.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 251.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 252.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 253.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 254.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 255.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 256.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 257.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 258.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 259.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 260.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 261.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 262.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 263.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 264.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 265.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 266.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 267.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 268.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 269.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 270.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 271.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 272.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 273.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 274.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 275.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 276.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 277.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 278.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 279.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 280.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame burning at alpha = 281.0000. Proceeding to the next iteration, with delta_alpha = 1.0
Flame extinguished at alpha = 282.0000. Restoring alpha = 281.0000 and trying delta_alpha = 0.02
Flame burning at alpha = 281.0200. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.0400. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.0600. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.0800. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.1000. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.1200. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.1400. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.1600. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.1800. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.2000. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.2200. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.2400. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.2600. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.2800. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.3000. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.3200. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.3400. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.3600. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.3800. Proceeding to the next iteration, with delta_alpha = 0.02
Error: Did not converge at n = 301
*******************************************************************************
CanteraError thrown by OneDim::timeStep:
Took maximum number of timesteps allowed (500) without reaching steady-state solution.
*******************************************************************************
Flame burning at alpha = 281.4000. Proceeding to the next iteration, with delta_alpha = 0.02
Error: Did not converge at n = 302
*******************************************************************************
CanteraError thrown by OneDim::timeStep:
Took maximum number of timesteps allowed (500) without reaching steady-state solution.
*******************************************************************************
Flame burning at alpha = 281.4200. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.4400. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.4600. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.4800. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.5000. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.5200. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.5400. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.5600. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.5800. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.6000. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.6200. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.6400. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.6600. Proceeding to the next iteration, with delta_alpha = 0.02
Flame burning at alpha = 281.6800. Proceeding to the next iteration, with delta_alpha = 0.02
Flame extinguished at alpha = 281.7000. Restoring alpha = 281.6800 and trying delta_alpha = 0.0004
Flame burning at alpha = 281.6804. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6808. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6812. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6816. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6820. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6824. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6828. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6832. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6836. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6840. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6844. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6848. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6852. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6856. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6860. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6864. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6868. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6872. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6876. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6880. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6884. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6888. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6892. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6896. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6900. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6904. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6908. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6912. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6916. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6920. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6924. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6928. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6932. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6936. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6940. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6944. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6948. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6952. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6956. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6960. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6964. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6968. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6972. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6976. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6980. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6984. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6988. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame burning at alpha = 281.6992. Proceeding to the next iteration, with delta_alpha = 0.0004
Flame extinguished at alpha = 281.6996. Restoring alpha = 281.6992 and trying delta_alpha = 8e-06
Flame burning at alpha = 281.6992. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6992. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6992. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6992. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6992. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6992. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6993. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6993. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6993. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6993. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6993. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6993. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6993. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6993. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6993. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6993. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6993. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6993. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6994. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6994. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6994. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6994. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6994. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6994. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6994. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6994. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6994. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6994. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6994. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6994. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6994. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6995. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6995. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6995. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6995. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6995. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6995. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6995. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6995. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6995. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6995. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6995. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6995. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6996. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6996. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6996. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6996. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6996. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame burning at alpha = 281.6996. Proceeding to the next iteration, with delta_alpha = 8e-06
Flame extinguished at alpha = 281.6996. Restoring alpha = 281.6996 and trying delta_alpha = 1.6e-07
Flame extinguished at alpha = 281.6996. Abortion criterion satisfied.
Results#
Print some parameters at the extinction point, after restoring the last burning solution
file_name, entry = names(f"extinction/{n_last_burning:04d}")
f.restore(file_name, entry)
print('----------------------------------------------------------------------')
print('Parameters at the extinction point:')
print('Pressure p={0} bar'.format(f.P / 1e5))
print('Peak temperature T={0:4.0f} K'.format(np.max(f.T)))
print('Mean axial strain rate a_mean={0:.2e} 1/s'.format(f.strain_rate('mean')))
print('Maximum axial strain rate a_max={0:.2e} 1/s'.format(f.strain_rate('max')))
print('Fuel inlet potential flow axial strain rate a_fuel={0:.2e} 1/s'.format(
f.strain_rate('potential_flow_fuel')))
print('Oxidizer inlet potential flow axial strain rate a_ox={0:.2e} 1/s'.format(
f.strain_rate('potential_flow_oxidizer')))
print('Axial strain rate at stoichiometric surface a_stoich={0:.2e} 1/s'.format(
f.strain_rate('stoichiometric', fuel='H2')))
----------------------------------------------------------------------
Parameters at the extinction point:
Pressure p=1.0000000000000007 bar
Peak temperature T=1552 K
Mean axial strain rate a_mean=1.58e+05 1/s
Maximum axial strain rate a_max=6.54e+05 1/s
Fuel inlet potential flow axial strain rate a_fuel=3.12e+05 1/s
Oxidizer inlet potential flow axial strain rate a_ox=1.01e+05 1/s
Axial strain rate at stoichiometric surface a_stoich=2.71e+05 1/s
Plot the maximum temperature over the maximum axial velocity gradient
plt.figure()
plt.semilogx(a_max, T_max)
plt.xlabel(r'$a_{max}$ [1/s]')
plt.ylabel(r'$T_{max}$ [K]')
plt.savefig(output_path / "figure_T_max_a_max.png")
plt.show()
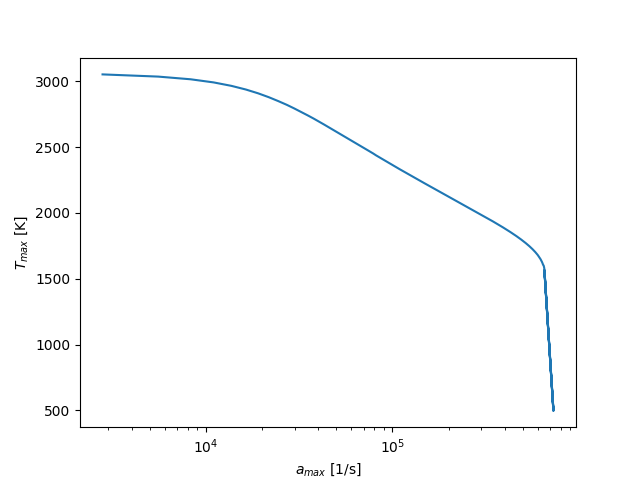
Total running time of the script: (1 minutes 16.909 seconds)